Due to the nature of how JavaScript works, you will most likely see an “Eliminate render-blocking resources” warning in speed testing tools. This means your JavaScript files slow down (block) the first paint of your WordPress site’s page when loading. This impacts both First Contentful Paint (FCP) and Largest Contentful Paint (LCP).
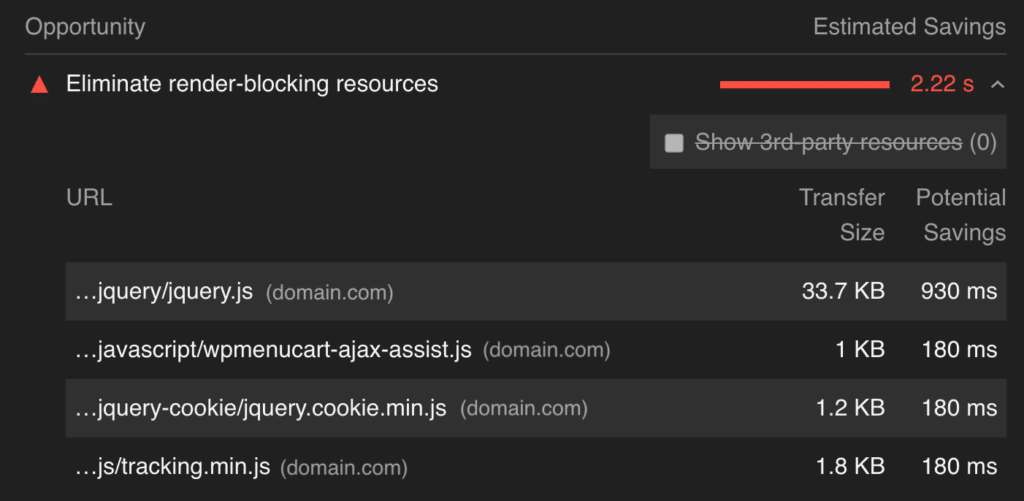
A great way to speed up the paint of a page is to defer all non-critical JavaScript. This means the JavaScript is downloaded during HTML parsing and will execute after the page has finished loading (when the parser has completed). Think of it like pushing all the JavaScript to the bottom of the waterfall. This is done by adding a defer
attribute on each JavaScript file.
<script type='text/javascript' src='https://domain.com/script.js' defer></script>
Deferring JavaScript will also fix the warning regarding avoid chaining critical requests.
Note: Deferred scripts are executed in the order they appear in the HTML document.
How to defer JavaScript with Perfmatters
You can enable JavaScript deferring in Perfmatters to fix render-blocking warnings and speed up the paint of a page. Follow the steps below.
There is no need to defer JavaScript multiple times. If you’re already deferring it with another plugin, then you can keep using that or switch over to Perfmatters.
Step 1
Click into the Perfmatters plugin settings.
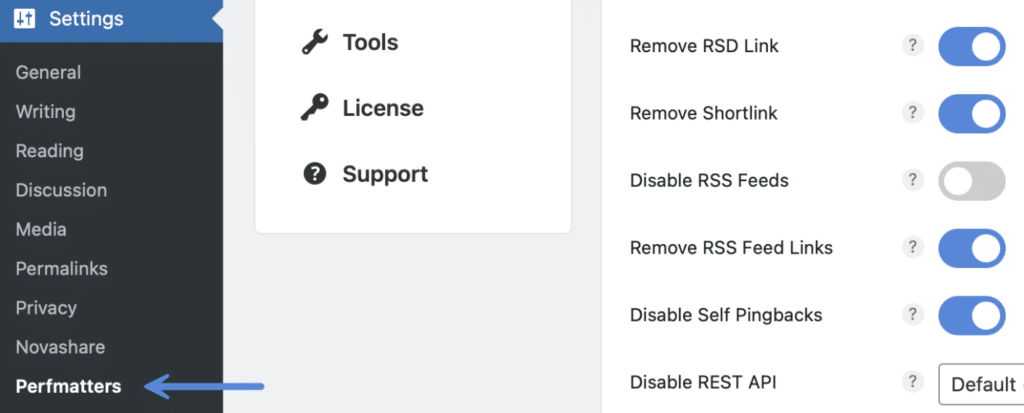
Step 2
Click on the “Assets” submenu.
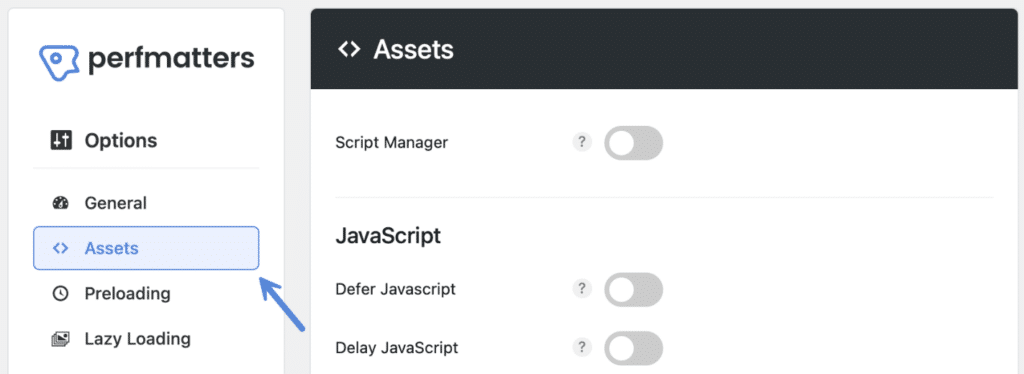
Step 3
Under the “JavaScript” section, toggle on “Defer JavaScript.” This adds a defer
attribute to all of your JavaScript files.

There is a check to see if the script already has a defer
attribute, so you don’t need to worry about it getting multiple attributes applied.
Step 4
Scroll down and click “Save Changes.”
Include jQuery
There is also an optional feature to “Include jQuery.” This allows jQuery core, which is part of WordPress, to be deferred. Note: This requires “Defer JavaScript” and “Advanced Options” to be toggled on.
Warning: We recommend testing this separately or leaving it off. Many plugins and themes require jQuery and deferring it might cause problems on your site.

How to add JavaScript deferral exclusions
Based on the order a JavaScript file or tag is needed, deferring it might cause problems. If needed, you can exclude it by the src URL.
Add JS exclusion by script
For JavaScript files, enter the name of the script into the “Exclude from Deferral” box. Such as script.js
(one per line).
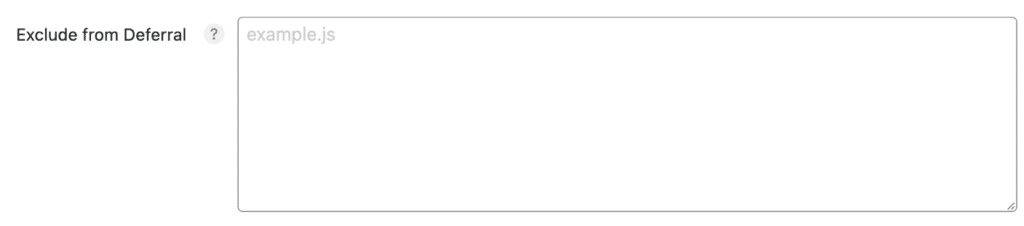
This should be unique, so if you have a generic file name, you can add a partial path to include the plugin’s folder on your site. Example: /generatepress/js/menu.min.js
Troubleshooting Deferral
If you think deferral is causing an issue, you can always uncheck the options above temporarily to test.
How to exclude a page/post from deferral
You can exclude JavaScript deferral on an individual post, page, or custom post type. In the editor, on the right-hand side uncheck “Defer JavaScript.” This will exclude the entire page from deferral. This can be useful for say a contact us page that might have more issues than the rest of the site.

Keep in mind that we automatically exclude specific URLs from optimizations when running WooCommerce (cart, checkout, account) for compatibility reasons. The Perfmatters meta box will not be visible when editing these pages.
Common exclusions
When using the Defer JavaScript option in Perfmatters, you might need to add an exclusion or two. The number is typically based on the complexity of the site.
Below are some common exclusions we typically see.
WordPress core
hooks.min.js i18n.min.js
perfmatters_defer_js filter
The perfmatters_defer_js filter
allows you to manipulate JS deferral. Below is an example of how to turn off defer on pages only.
add_filter('perfmatters_defer_js', function($defer) {
if(is_singular('page')) {
return false;
}
return $defer;
});